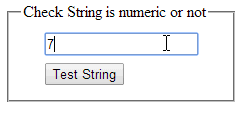
Description: While working on
asp.net project i got the requirement to test whether the entered string is
number or not? The solution is very easy and i am going to share two solutions
for this with all so that other developers can implement it in their project
easily whenever required.
Check the first solution by visiting
the link "Check whether a string is numeric or not in Asp.net" and second you will get in this article using regular
expression.
In previous articles i explained how to Get city, state and country based on zip code using Google map API and Bind state categories and cities sub categories in single dropdownlist and Add meta description,meta keywords tags from code behind and Drag & drop to upload multiple files using AjaxFileUpload like Facebook
Implementation: Let's create a
demo small web application to test the string is number or not.
Asp.Net C# Section
- In the <Form> tag of the design page(default.aspx) design the page as:
<div>
<fieldset style="width:200px;">
<legend>Check String is numeric or not</legend>
<center>
<table>
<tr>
<td>
<asp:TextBox ID="txtTest"
runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Button ID="btnTest"
runat="server"
onclick="btnTest_Click"
Text="Test
String" /><br />
<asp:Label ID="lblStatus"
runat="server"></asp:Label>
</td>
</tr>
</table>
</center>
</fieldset>
</div>
- In the code behind file(default.aspx.cs) write the code as:
protected void btnTest_Click(object
sender, EventArgs e)
{
if (IsNumeric(txtTest.Text.Trim()))
{
lblStatus.Text = txtTest.Text + " is
Numeric";
lblStatus.ForeColor = System.Drawing.Color.Green;
}
else
{
lblStatus.Text = txtTest.Text + " is
not Numeric";
lblStatus.ForeColor = System.Drawing.Color.Green;
}
}
System.Text.RegularExpressions.Regex reg = new
System.Text.RegularExpressions.Regex(@"^[0-9]+$");
public bool
IsNumeric(String str)
{
try
{
if (string.IsNullOrEmpty(str))
{
return false;
}
if (!reg.IsMatch(str))
{
return false;
}
}
catch (Exception
ex)
{
lblStatus.Text = "Error occured :
" + ex.Message.ToString();
lblStatus.ForeColor = System.Drawing.Color.Red;
}
return false;
}
Asp.Net VB section:
- In the <Form> tag of the design page(default.aspx) design the page as:
<div>
<fieldset style="width:200px;">
<legend>Check String is numeric or not</legend>
<center>
<table>
<tr>
<td>
<asp:TextBox ID="txtTest"
runat="server"></asp:TextBox>
</td>
</tr>
<tr>
<td>
<asp:Button ID="btnTest"
runat="server"
Text="Test
String" /><br />
<asp:Label ID="lblStatus"
runat="server"></asp:Label>
</td>
</tr>
</table>
</center>
</fieldset>
</div>
- In the code behind file (default.aspx.vb) write the code as:
Protected Sub btnTest_Click(sender As
Object, e As
System.EventArgs) Handles
btnTest.Click
If IsNumeric(txtTest.Text.Trim()) Then
lblStatus.Text = txtTest.Text + " is
Numeric"
lblStatus.ForeColor = System.Drawing.Color.Green
Else
lblStatus.Text = txtTest.Text + " is
not Numeric"
lblStatus.ForeColor = System.Drawing.Color.Green
End If
End Sub
Private reg As New System.Text.RegularExpressions.Regex("^[0-9]+$")
Public Function
IsNumeric(str As [String])
As Boolean
Try
If String.IsNullOrEmpty(str)
Then
Return False
End If
If Not
reg.IsMatch(str) Then
Return False
End If
Catch ex As Exception
lblStatus.Text = "Error occured :
" & ex.Message.ToString()
lblStatus.ForeColor = System.Drawing.Color.Red
End Try
Return False
End Function
Now over to you:
" I hope you have got How to Check whether entered string is numeric or not in Asp.Net using regular expression and If you like my work; you can appreciate by leaving your comments, hitting Facebook like button, following on Google+, Twitter, Linked in and Pinterest, stumbling my posts on stumble upon and subscribing for receiving free updates directly to your inbox . Stay tuned and stay connected for more technical updates."
If you have any question about any post, Feel free to ask.You can simply drop a comment below post or contact via Contact Us form. Your feedback and suggestions will be highly appreciated. Also try to leave comments from your account not from the anonymous account so that i can respond to you easily..