Introduction: While creating user input form e.g.
registration where user inputs the details like Name, email address, password
and confirm password etc. we have to implement validations on the form so that
incorrect data could not be submitted to server. Asp.net provides Validation controls like CompareValidator,
CustomValidator, RangeValidator, RegularExpressionValidator,
RequiredFieldValidator etc to apply validations on asp.net web page.
In this example I have demonstrated 3 different ways to use CompareValidator control.
In previous articles i explained How to use RequiredFieldValidator validation control with example in asp.net and RegularExpressionValidator and RangeValidator and CustomValidator and JavaScript validation in asp.net website and Jquery form validations in asp.net.
Now in this
article I will explain with example How to use CompareValidator control to compare the data in two TextBoxes e.g. Password
TextBox and Confirm password TextBox should be same before submitting to server
for processing otherwise error message will be displayed to the user.
In this example I have demonstrated 3 different ways to use CompareValidator control.
Case 1: If you want to show the
validation failure message near to text box for which the validation failed as
shown in figure below:
then In the design
page(.aspx) Place two TextBox controls for entering Password and Confirm
Password and a Button control for Submitting . Also place a CompareValidator validation
controls from validation category from the visual studio toolbox as shown in
figure below:
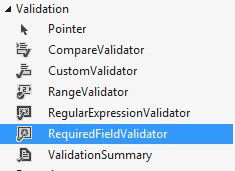
<fieldset style="width:450px;">
<legend>CompareValidator
example in asp.net</legend>
<table>
<tr>
<td>Password:
<span style="color:red;">*</span></td>
<td>
<asp:TextBox ID="txtPwd" runat="server" TextMode="Password"></asp:TextBox>
</td>
<td>
</td>
</tr>
<tr>
<td>Confirm
Password: <span style="color:red;">*</span></td>
<td>
<asp:TextBox ID="txtConfirmPwd" runat="server" TextMode="Password"></asp:TextBox>
</td>
<td><asp:CompareValidator ID="cmpPwd" runat="server" ControlToCompare="txtConfirmPwd" ControlToValidate="txtPwd" ErrorMessage="Password
didn't match" ForeColor="#FF3300" SetFocusOnError="True" ></asp:CompareValidator></td>
</tr>
<tr>
<td> </td>
<td>
<asp:Button ID="btnSubmit" runat="server" Text="Submit" />
</td>
</tr>
</table>
</fieldset>
Case 2: If you want the validation failure
message to show in pop up as shown in figure below:
then change the Display
property of the CompareValidator validation controls to None and place a
ValidationSummary control from the same validation category from the
visual studio toolbox. Set the ShowMessageBox property to true and
ShowSummary to false as shown below :
<fieldset style="width:450px;">
<legend>CompareValidator
example in asp.net</legend>
<table>
<tr>
<td>Password:
<span style="color:red;">*</span></td>
<td>
<asp:TextBox ID="txtPwd" runat="server" TextMode="Password"></asp:TextBox>
</td>
<td>
</td>
</tr>
<tr>
<td>Confirm
Password: <span style="color:red;">*</span></td>
<td>
<asp:TextBox ID="txtConfirmPwd" runat="server" TextMode="Password"></asp:TextBox>
</td>
<td><asp:CompareValidator ID="cmpPwd" runat="server" ControlToCompare="txtConfirmPwd" ControlToValidate="txtPwd" ErrorMessage="Password
didn't match" ForeColor="#FF3300" SetFocusOnError="True" Display="None" ></asp:CompareValidator></td>
</tr>
<tr>
<td> </td>
<td>
<asp:Button ID="btnSubmit" runat="server" Text="Submit" />
</td>
<td> </td>
</tr>
<tr>
<td colspan="3">
<asp:ValidationSummary ID="ValidationSummary1" runat="server" ForeColor="#FF3300" HeaderText="Please
correct the following:" ShowMessageBox="True" ShowSummary="False" />
</td>
</tr>
</table>
</fieldset>
Case 3: If you want to show validation failure
message in a separate place as shown in figure below :
then Set the ShowMessageBox property
to false and ShowSummary property to true as shown below.
<asp:ValidationSummary ID="ValidationSummary1" runat="server" ForeColor="#FF3300" HeaderText="Please
correct the following:" ShowMessageBox="false" ShowSummary="true"/>
Now over to you:
"If you like my work; you can appreciate by leaving your comments,
hitting Facebook like button, following on Google+, Twitter, Linked in and
Pinterest, stumbling my posts on stumble upon and subscribing for receiving
free updates directly to your inbox . Stay tuned and stay connected for more
technical updates."
If you have any question about any post, Feel free to ask.You can simply drop a comment below post or contact via Contact Us form. Your feedback and suggestions will be highly appreciated. Also try to leave comments from your account not from the anonymous account so that i can respond to you easily..