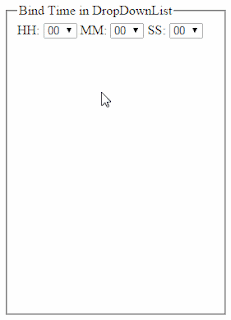
In previous article i explained How to Populate time in dropdownlist with interval of minutes or hours dynamically and Populate multiple dropdownlist from same datasource in single database calland Fill country, state and cities in dropdownlist and Bind state categories and cities sub categories in single dropdownlist and Ajax cascadingdropdown to fill countries, states and cities in dropdownlist
Description: While working on Asp.Net project I got the
requirement to fill hours, minutes and seconds in dropdownlist. There are
numerous ways to do so but adopted an optimized way. As we know we need to fill
from 0 up to 23 in hours and up to 59 in both minute and seconds dropdownlist.
So we can loop through 0 to 59 and fill up to 23 in hours dropdownlist based on condition
and upto full 59 in minutes and seconds dropdownlist as mentioned in the code
below.
Implementation: Let’s
create a sample web page to demonstrate the concept
HTML source
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
</head>
<body>
<form id="form1" runat="server">
<div>
<fieldset
style="width:
240px;
height:
360px;">
<legend>Bind Time in DropDownList</legend>
HH:
<asp:DropDownList ID="ddlHours" runat="server" />
MM:
<asp:DropDownList ID="ddlMinutes" runat="server" />
SS:
<asp:DropDownList ID="ddlSeconds" runat="server" />
</fieldset>
</div>
</form>
</body>
</html>
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
BindTime();
}
}
private void BindTime()
{
List<string> hours = new List<string>();
List<string> minutes = new List<string>();
List<string> seconds = new List<string>();
for (int i = 0; i <= 59; i++)
{
if (i < 24)
{
hours.Add(i.ToString("00"));
}
minutes.Add(i.ToString("00"));
seconds.Add(i.ToString("00"));
}
ddlHours.DataSource = hours;
ddlHours.DataBind();
ddlMinutes.DataSource = minutes;
ddlMinutes.DataBind();
ddlSeconds.DataSource = seconds;
ddlSeconds.DataBind();
}
Protected Sub Page_Load(sender As Object,
e As EventArgs)
Handles
Me.Load
If Not IsPostBack Then
BindTime()
End If
End Sub
Private Sub BindTime()
Dim hours As New List(Of String)()
Dim minutes As New List(Of String)()
Dim seconds As New List(Of String)()
For i As Integer = 0 To 59
If i < 24 Then
hours.Add(i.ToString("00"))
End If
minutes.Add(i.ToString("00"))
seconds.Add(i.ToString("00"))
Next
ddlHours.DataSource = hours
ddlHours.DataBind()
ddlMinutes.DataSource = minutes
ddlMinutes.DataBind()
ddlSeconds.DataSource = seconds
ddlSeconds.DataBind()
End Sub
Now over to you:
A blog is nothing without reader's feedback and comments. So please provide your valuable feedback so that i can make this blog better and If you like my work; you can appreciate by leaving your comments, hitting Facebook like button, following on Google+, Twitter, Linkedin and Pinterest, stumbling my posts on stumble upon and subscribing for receiving free updates directly to your inbox . Stay tuned and stay connected for more technical updates.
If you have any question about any post, Feel free to ask.You can simply drop a comment below post or contact via Contact Us form. Your feedback and suggestions will be highly appreciated. Also try to leave comments from your account not from the anonymous account so that i can respond to you easily..